File Handling in Python with example
In this article, we'll learn file handling in Python. Topics covered in this tutorial are create a file in Python, open a file in Python, read and write files in Python, and Python file functions.
Python is the most popular language in the world. This tutorial is about file handling in Python and how to work with files. In this tutorial, I've covered the following topics
- What is a file?
- What is file handling in Python?
- Types of files
- Handling operation on files
- Python file methods
- How to open files in Python
- How to read files in Python
- How to write files in Python
- How to append files in Python
If you're new to Python, please go through the below articles.
Let's explore each and every one, one by one.
What is a file?
It is a container of user information and stores data permanently. It is is located on the computer's hard drive, which is non-volatile memory in nature.
What is file handling in Python?
Python provides inbuilt functionally to work with files including open files, read and write files, and manipulate files. The process of working with files is called file handling.
File handling concepts of Python is easy to learn and understand. Each and every line of a file is terminated with a special character which is called End of Line (EOL) like “,","-","\n" and so on.
Types of files in Python
These are the two types of files in Python we will focus in this tutorial.
- Text file
- Binary file
Text file:
Text files contains plain text and does not contains encoded data and can be opened in any text editor. Text files are stored in a human-readable format (usually ASCII).
Example:
txt, rtf, csv, tsv, js, java,c, html, xml, json, css etc.
Binary files:
It is a special type of file which is used by computer system in the form of computer-readable form, not human readable. The content of binary files are 0s and 1s that made instructions read by the computers. All executable programs are stored in binary files. Binary files are very smaller that a text files.
In this article we will see only File Operation on Text Files.
Python file handling operations on text files:
The 6 common operations we usually perform on text files are the following:
- Open a text file
- Read a text file
- Write to a text file
- Close a text file
- Rename a text file
- Delete a text file
Example:
Working with a file in Python is no different than we use a physical file or a diary in our daily lives. First, we grab the file from its location, open the file, read or write to it or remove something, and then we close it. Same concept applies to digital files.
Open a file in Python
Python has an in-built function called open () to open a text file in Python.
Syntax
openfile_object = open(fiename, mode)
Note:
The filename is the location of the file or the name of file (with extension) that we want to open.
A file can be opened for a specific operation or operations that is managed by using file modes. Python provides different types of modes. They are as given below.
- ‘r' – Read Mode: This mode is used for read data from the files.
- ‘w' – Write Mode: This mode is used for write data into the file or modify it. Write mode always overwrites the data into the files.
- ‘a' – Append Mode: This mode is used to append data to the file. Append mode will always append the data into the EOF (End of File).
- ‘r+' – Read or Write Mode: This mode is used to read or write the data from the same file.
- ‘a+' – Append or Read Mode: This mode is used to read data from the file or append the data into the same file.
Let’s start,
Step 1.
First, let's create a sample text file with the name of "PythonText.txt" as shown below. You can create the text file in Notepad and save it.
First, let's create a sample text file with the name of "PythonText.txt" as shown below. You can create the text file in Notepad and save it.

Listing 1.
Step 2.
Create a python file with name "test.py" in your python editor or any IDE you're using to write Python programs.
Create a python file with name "test.py" in your python editor or any IDE you're using to write Python programs.
Step 3.
Open text file (PythonText.txt) with read-only mode (r).
Open text file (PythonText.txt) with read-only mode (r).
Python provides 3 function to read the content of the file. They are as given below.
- Read (): This function reads whole content of the file.
- Read(size): This function reads specific number of sizes starting from the files.
- Readline (): This function reads a single line from the file with newline at the end
- Readlines (): This function returns a list containing all the lines in the file.
- Read(): This function is used to read whole file at a time.
Let's explore one by one.
Read ():
This function reads whole content of the file.
Code:
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","r")
- print(read_file.read())
Here, read () function reads whole file content and print command prints it.
Output:

Listing 2
If we want to print specific number of characters from the file then use below code.
Read (size):
This function reads specific number of sizes from the beginning of the file.
Syntax:
read (number of characters)
Code
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","r")
- print(read_file.read(22))
Output:

Listing 3.
Readline():
This function reads a single line from the file with newline at the end.
Code
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","r")
- print(read_file.readline())
Output

Listing 4.
Readlines():
This function returns a list containing all the lines in the file.
Syntax:
readlines()
Code
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","r")
- print(read_file.readlines())
Output
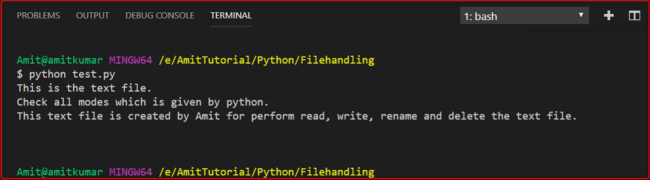
Listing 5.
How to read specific row form the files
Code
- line_number = eval (input("Enter number of line for read: "))
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","r")
- start_line=1
- for file_lines in read_file:
- if start_line==line_number:
- print(file_lines)
- break
- else:
- start_line =start_line + 1
If user enter line number 1.
Output

Listing 6.
How to write text file in Python
There are 2 ways to write text to file in Python.
Write single line to text file in Python
You can create a new file or write to an existing file. The write operations are controlled by the write file mode. If you want to create and write to a new file, with “w” (open) mode. The “w” mode will delete any previous existing file content and create a new file to write.
Code:
- line =input("Enter text for write in the file: ")
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","w")
- # write a line to output file
- read_file.write(line)
- read_file.write("\n")
- read_file.close()
Before execute the command, you can see in our file that already contains some text.

Listing 7.
After executing the code.
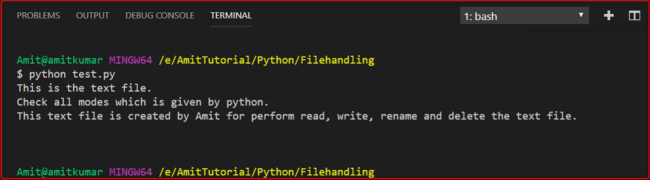
As you can see, we have multiple lines on the previous file. But after executing the code, old content is deleted and new content is written to the file. See our new file's content:
Output

Listing 8.
Write multiple lines in a text file
Code
- txtList = ["One-1", "Two-2", "Three-3", "Four-4", "Five-5"]
Now write this value into the existing file (PythonText.Text).
Open the file with append mode and use the following code.
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","a")
- # write a line to output file
- txtList = ["One-1", "Two-2", "Three-3", "Four-4", "Five-5"]
- for line in txtList:
- # write line to output file
- read_file.write(line)
- read_file.write("\n")
- read_file.close()
Output:

Listing 9.
Append to File in Python:
To append a file, we must open file in 'a+' mode so that we will have access to both the append as well as write modes.
Example:
Code
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","a")
Here I have opened file in the a+ mode that means append and write mode.
Code:
- read_file= open("E:\AmitTutorial\Python\Filehandling\PythonText.txt","a+")
- read_file.write("Six-6")
- read_file.write("\n")
- read_file.close()
Output

Listing 10.
File Rename in Python
Python provides an "os" module, which contains some in-built methods for performing other file operations.
rename()
This function is used for rename a file.
This rename() method accepts two arguments. The first argument is the original file with its location and the second is the new file with its location.
Syntax:
os.rename(current_file_name, new_file_name)
example
- import os
- os.rename("E:\AmitTutorial\Python\Filehandling\PythonText.txt", "E:\AmitTutorial\Python\Filehandling\PythonTextfile.txt")
Output

Listing 11.
Conclusion: In this article we learned file operations on text files. In my next article, I will cover file handling operations on binary files.
I hope you liked this article.
Leave a Comment